This tutorial uses example Python codes to show 2 methods to read a text (txt) file into the Python programming environment. The following is the screenshot of the txt file.
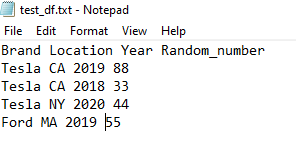
Method 1: Use read_csv() function to read txt
You can use read_csv()
function to read txt files as well. The basic syntax structure is as follows. I also provide example Python code below.
pd.read_csv("file_name.txt", sep=" ")
import pandas as pd
#read the txt file using pd.read_csv()
test_df = pd.read_csv("test_df.txt", sep=" ")
#print out the dataframe
print(test_df)
Brand Location Year Random_number 0 Tesla CA 2019 88 1 Tesla CA 2018 33 2 Tesla NY 2020 44 3 Ford MA 2019 55
By default, it assumes there is header. If no, you can tell the function that there is no header. The following shows how it would look like. Obviously, this txt file actually has a header.
import pandas as pd
#read the txt file using pd.read_csv()
test_df = pd.read_csv("test_df.txt", sep=" ",header=None)
#print out the dataframe
print(test_df)
0 1 2 3 0 Brand Location Year Random_number 1 Tesla CA 2019 88 2 Tesla CA 2018 33 3 Tesla NY 2020 44 4 Ford MA 2019 55
Method 2: Use read_table() function to read txt files
You can also use read_table()
function to read txt files as well. The basic syntax structure is as follows.
pd.read_table("file_name.txt", sep=" ")
import pandas as pd
#read the txt file using pd.read_table()
test_df = pd.read_table("test_df.txt", sep=" ")
#print out the dataframe
print(test_df)
Brand Location Year Random_number 0 Tesla CA 2019 88 1 Tesla CA 2018 33 2 Tesla NY 2020 44 3 Ford MA 2019 55