This tutorial shows how you can convert dataframe columns to vectors in R with examples. The following shows the two methods to do it.
Method 1:
vector<-df$column
Method 2:
vector<-df[ , ‘column’]
Examples of Convert Dataframe columns to Vectors in R
Example 1 (Method 1)
The following is the R code to read a CSV file from GitHub as a dataframe and then select the column of write as a vector. Then, it prints out the saved vector.
# Import the data from GitHub hsbdemo <- read.csv(file = 'https://raw.githubusercontent.com/TidyPython/SPSS/main/hsbdemo.csv') # save the dataframe column to a vector writing<-hsbdemo$write # print out the vector print(writing)
Output:
[1] 35 33 39 37 31 36 36 31 41 37 44 33 31 44 35 44 46 46 46 49 39 44 47 44 37 38 49 44 41 50 44 [32] 39 40 46 41 39 33 59 41 47 31 42 55 40 44 54 46 54 42 49 41 41 44 57 52 49 41 57 62 33 54 40 [63] 52 57 49 46 57 49 52 53 54 57 41 52 57 54 52 52 44 46 49 54 52 44 49 46 54 39 59 45 52 54 44 [94] 50 62 59 52 52 46 41 52 52 55 41 49 59 54 54 59 55 62 54 54 54 59 57 61 52 59 62 60 59 65 61 [125] 59 59 59 59 65 57 59 49 49 59 62 59 54 63 43 54 52 57 57 57 61 62 62 65 57 59 59 62 65 62 62 [156] 62 54 62 65 62 67 65 60 59 59 59 65 62 65 59 59 61 65 67 65 65 67 65 62 52 63 59 65 59 67 67 [187] 60 67 62 54 65 62 59 60 63 65 63 67 65 62
Example 2 (Method 2)
The following uses the dataset but with Method 2.
# Import the data from GitHub hsbdemo <- read.csv(file = 'https://raw.githubusercontent.com/TidyPython/SPSS/main/hsbdemo.csv') # save the dataframe column to a vector writing<-hsbdemo[ ,'write'] # print out the vector print(writing)
Output:
[1] 35 33 39 37 31 36 36 31 41 37 44 33 31 44 35 44 46 46 46 49 39 44 47 44 37 38 49 44 41 50 44 [32] 39 40 46 41 39 33 59 41 47 31 42 55 40 44 54 46 54 42 49 41 41 44 57 52 49 41 57 62 33 54 40 [63] 52 57 49 46 57 49 52 53 54 57 41 52 57 54 52 52 44 46 49 54 52 44 49 46 54 39 59 45 52 54 44 [94] 50 62 59 52 52 46 41 52 52 55 41 49 59 54 54 59 55 62 54 54 54 59 57 61 52 59 62 60 59 65 61 [125] 59 59 59 59 65 57 59 49 49 59 62 59 54 63 43 54 52 57 57 57 61 62 62 65 57 59 59 62 65 62 62 [156] 62 54 62 65 62 67 65 60 59 59 59 65 62 65 59 59 61 65 67 65 65 67 65 62 52 63 59 65 59 67 67 [187] 60 67 62 54 65 62 59 60 63 65 63 67 65 62
Difference between vectors and columns in R
Note that, hsbdemo[ ,'write']
is different from hsbdemo['write']
.
# save the dataframe column as writing2 writing2<-hsbdemo['write'] # print out writing2 print(writing2)
The following is the output, and it shows how writing2
looks like.
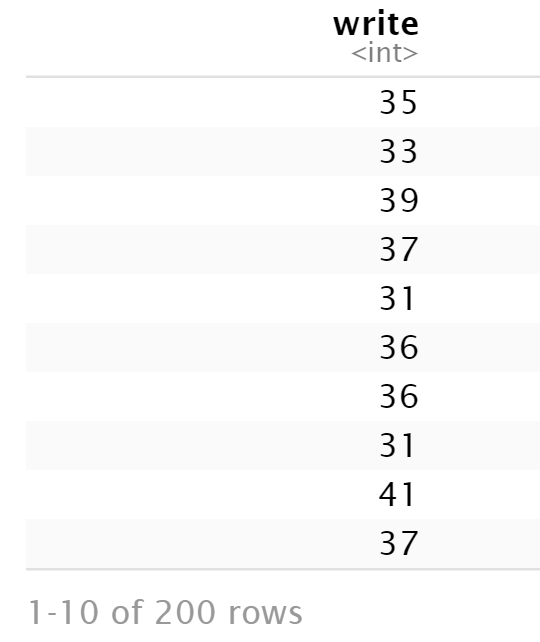
The following R code checks whether writing2
is a dataframe and the output shows that it is still a dataframe, even just one column.
# check if writing2 is a dataframe is.data.frame(writing2) # The following is the output of is.data.frame(writing2) [1] TRUE
To conclude, this tutorial provides two examples to convert dataframe columns to vectors in R with examples. Further, this tutorial shows the difference between df[,’column_name’] and df[‘column_name’].
Further Reading
In the following tutorial of t-test, there is a need to select vectors in the R code. You can see that convert dataframe columns to vectors in R is pretty common.
Further, opposite to this tutorial, you can also combine vectors into a dataframe in R. The following is the tutorial.