This tutorial shows how to create OpenAI website style illustrations using Python Turtle graphics. The following includes two examples.
Example 1: Parallel Lines
The following is the first example of drawing an OpenAI-style figure using Python Turtle. If you run the code, you will get the following figure, which includes some parallel lines with different gray shapes.
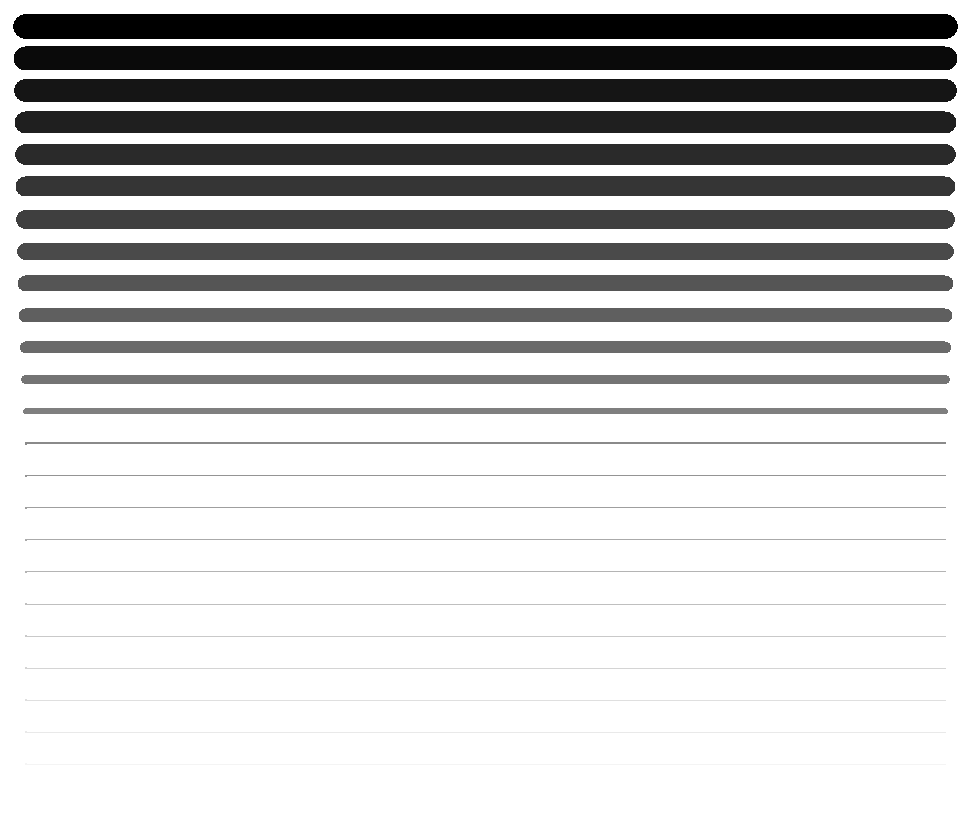
import turtle
# Set up the turtle screen
screen = turtle.Screen()
# Create turtle objects
turtles = []
# Set up the turtles
line_height = screen.window_height() - 40
num_lines = int(line_height / 30) # Adjust the distance between lines as needed
# Calculate the distance between lines
distance_between_lines = line_height / (num_lines - 1)
# Calculate the maximum line width at the top
max_line_width = 25
# Set the speed of decreasing width (increase the value for slower decrease)
width_decrease_speed = 1.1
# Loop to create multiple parallel lines
for i in range(num_lines):
# Create a turtle object
new_turtle = turtle.Turtle()
# Calculate the distance from the top
distance_from_top = i * distance_between_lines
# Calculate the line width based on the distance from the top
denominator = max(1, line_height - (width_decrease_speed * distance_from_top)) # Add a small offset to avoid division by zero
line_width = max_line_width - 0.7 *(max_line_width / denominator) * distance_from_top
# Ensure line_width is always positive
line_width = max(line_width, 0)
# Set the turtle width and speed
new_turtle.width(line_width)
new_turtle.speed(0)
# Calculate the grayscale color value based on the line index
grayscale_value = int((255 / (num_lines - 1)) * i)
# Convert the grayscale value to hexadecimal format
hex_color = "#{:02x}{:02x}{:02x}".format(grayscale_value, grayscale_value, grayscale_value)
# Set the turtle color
new_turtle.color(hex_color)
# Add the turtle to the list
turtles.append(new_turtle)
# Set the initial position for the lines
start_x = -screen.window_width() / 2 + 20
start_y = screen.window_height() / 2 - 20
# Set the shape of the turtle to square
screen.register_shape("square", ((-0.5, -0.5), (-0.5, 0.5), (0.5, 0.5), (0.5, -0.5)))
for turtle_obj in turtles:
turtle_obj.shape("square")
# Loop to draw multiple parallel lines
for i, turtle_obj in enumerate(turtles):
# Move the turtle to the starting position of the line
turtle_obj.penup()
turtle_obj.setpos(start_x, start_y - i * distance_between_lines)
turtle_obj.pendown()
# Set the orientation of the turtle to draw straight lines
turtle_obj.setheading(0)
# Calculate the line length based on the width
line_length = screen.window_width() - 40
# Draw the line using forward and backward movements
turtle_obj.forward(line_length)
turtle_obj.backward(line_length)
# Close the turtle graphics window
turtle.done()
Example 2: Random Squares
In the following example, we are going to draw random squares in different rows with different levels of gray.
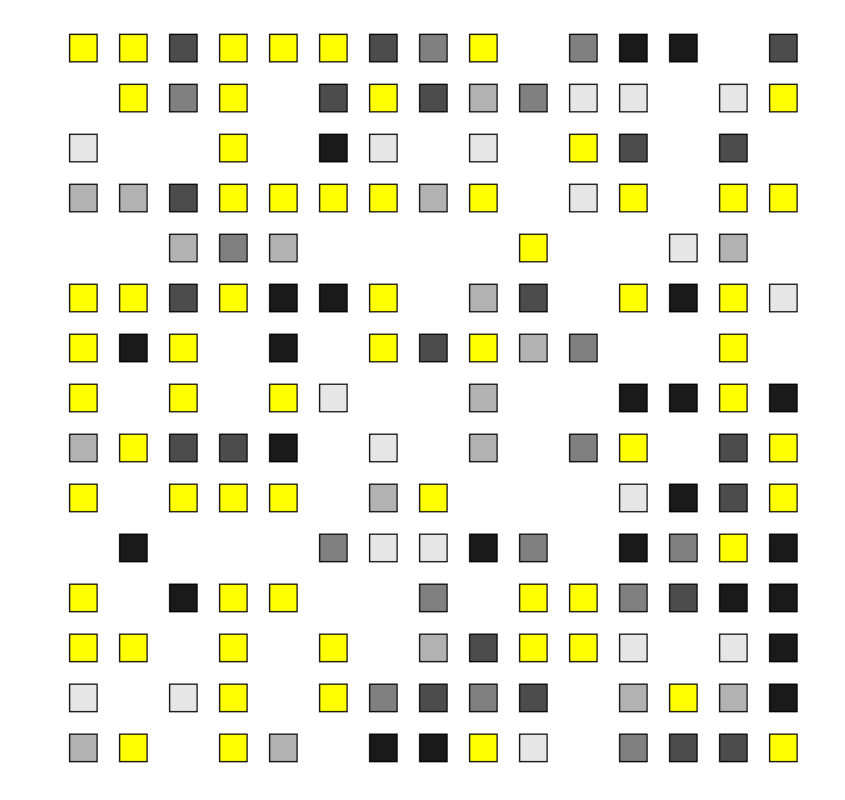
import turtle
import random
# Set up the turtle screen
screen = turtle.Screen()
screen.bgcolor("white")
# Create a turtle instance
t = turtle.Turtle()
t.shape("turtle")
t.speed(0)
# Function to draw a square at a given location and size
def draw_square(x, y, size, color):
t.penup()
t.goto(x - size / 2, y - size / 2)
t.pendown()
t.fillcolor(color)
t.pensize(0) # Set the pen size to 0 (no outline)
t.begin_fill()
for _ in range(4):
t.forward(size)
t.right(90)
t.end_fill()
# Number of rows and squares per row
num_rows = 15
squares_per_row = 15
# Distance between squares and rows
square_spacing = 40
row_spacing = 40
# Starting position of the first row
start_x = -square_spacing * (squares_per_row - 1) / 2
start_y = (num_rows - 1) * row_spacing / 2
# Gray levels
gray_levels = [0.1, 0.3, 0.5, 0.7, 0.9]
# Draw squares in rows
for row in range(num_rows):
y = start_y - row * row_spacing
for col in range(squares_per_row):
x = start_x + col * square_spacing
# Randomly decide whether to remove the square
if random.random() < 0.3: # Adjust the probability as desired
continue # Skip drawing the square
# Determine the color for the square
if random.random() < 0.4: # Adjust the probability as desired
color = "yellow" # Use yellow color
else:
gray_level = random.choice(gray_levels)
color = (gray_level, gray_level, gray_level) # Use gray color
draw_square(x, y, 22, color) # Increase square size to 20
# Hide the turtle cursor
t.hideturtle()
# Update the screen
screen.update()
# Exit on click
turtle.done()